Actson: a reactive (or non-blocking, or asynchronous) JSON parser
Today, I’m happy to announce the very first version of Actson, a reactive (sometimes referred to as non-blocking or asynchronous) JSON parser. Actson is event-based and can be used together with reactive libraries/tool-kits such as RxJava or Vert.x. The library is very small and has no dependencies. It only requires Java 7 (or higher).
Download the Actson binaries from search.maven.org or the source code from the GitHub repository. Actson is released under the MIT license.
Why another JSON parser?
- Non-blocking. Other JSON parsers use blocking I/O (i.e. they read from an
InputStream
). If you want to develop a reactive application, you should use non-blocking I/O (see the Reactive Manifesto). - Big Data. Most parsers read the full JSON text into memory to map it to a POJO, for example. Actson can handle arbitrarily large JSON text. It is event-based and can be used for streaming.
- GeoRocket. Actson was primarily developed for GeoRocket, a high-performance reactive data store for geospatial files. We use Aalto XML to parse XML in a non-blocking way and we needed something similar for GeoRocket’s GeoJSON support.
Usage
The JSON parser gets its input data from a feeder. Actson uses a push-pull approach: Push data into the feeder until it is full. Then, call the parser until it wants more data. Repeat this process until the whole JSON text has been parsed.
The following snippet demonstrates how you can use the parser sequentially.
// JSON text to parse
byte[] json = "{\"name\":\"Elvis\"}".getBytes(StandardCharsets.UTF_8);
JsonParser parser = new JsonParser(StandardCharsets.UTF_8);
int pos = 0; // position in the input JSON text
int event; // event returned by the parser
do {
// feed the parser until it returns a new event
while ((event = parser.nextEvent()) == JsonEvent.NEED_MORE_INPUT) {
// provide the parser with more input
pos += parser.getFeeder().feed(json, pos, json.length - pos);
// indicate end of input to the parser
if (pos == json.length) {
parser.getFeeder().done();
}
}
// handle event
System.out.println("JSON event: " + event);
if (event == JsonEvent.ERROR) {
throw new IllegalStateException("Syntax error in JSON text");
}
} while (event != JsonEvent.EOF);
Reactive, non-blocking examples
Find more complex and elaborate usage examples in the GitHub repository.
Similar libraries
- Jackson has a streaming API that
produces JSON tokens/events. However, it uses blocking I/O because it reads
from an
InputStream
. - Aalto XML is similar to Actson but parses XML instead of JSON.
Acknowledgments
The event-based parser code and the JSON files used for testing are largely based on the file JSON_checker.c and the JSON test suite from JSON.org originally released under this license (basically MIT license).
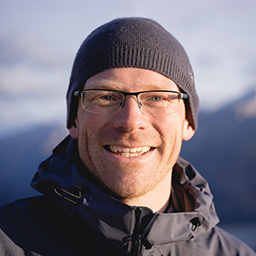
Posted by Michel Krämer
on 19 August 2016
Next post
Actson 1.2.0 with RFC 7159 compliance
The next version of my reactive JSON parser is available! The update includes RFC 7159 compliance, performance improvements, and minor bug fixes. The library is small and fast. Updating is recommended for all users.
Previous post
gradle-download-task 3.0.0
I’m happy to announce a new version of the popular gradle-download-task. The plugin’s core has been completely rewritten. It now supports proxy servers and creates target directories automatically. Updating is recommended for all users.
Related posts
10 recipes for gradle-download-task
gradle-download-task is a Gradle plugin that allows you to download files during the build process. This post summarizes common patterns and use cases of gradle-download-task and provides useful tips and tricks.
GeoRocket 1.3.0
Only two months after the last big release of GeoRocket, we’ve published a new version featuring highlights such as low-latency optimistic merging and progress display during import. Also, a few minor bugs have been fixed.
5 anti-spam measures for phpBB 3.0
Discussion boards powered by phpBB are regularly targeted by spammers. Version 3 has introduced an improved Captcha but there are more possibilities to reduce spam. In this article, I present five useful and effective measures.